cart
The cart
template renders the /cart
page, which provides an overview of the contents of a customer’s cart. The overview is typically shown in a table format with a row for each line item.
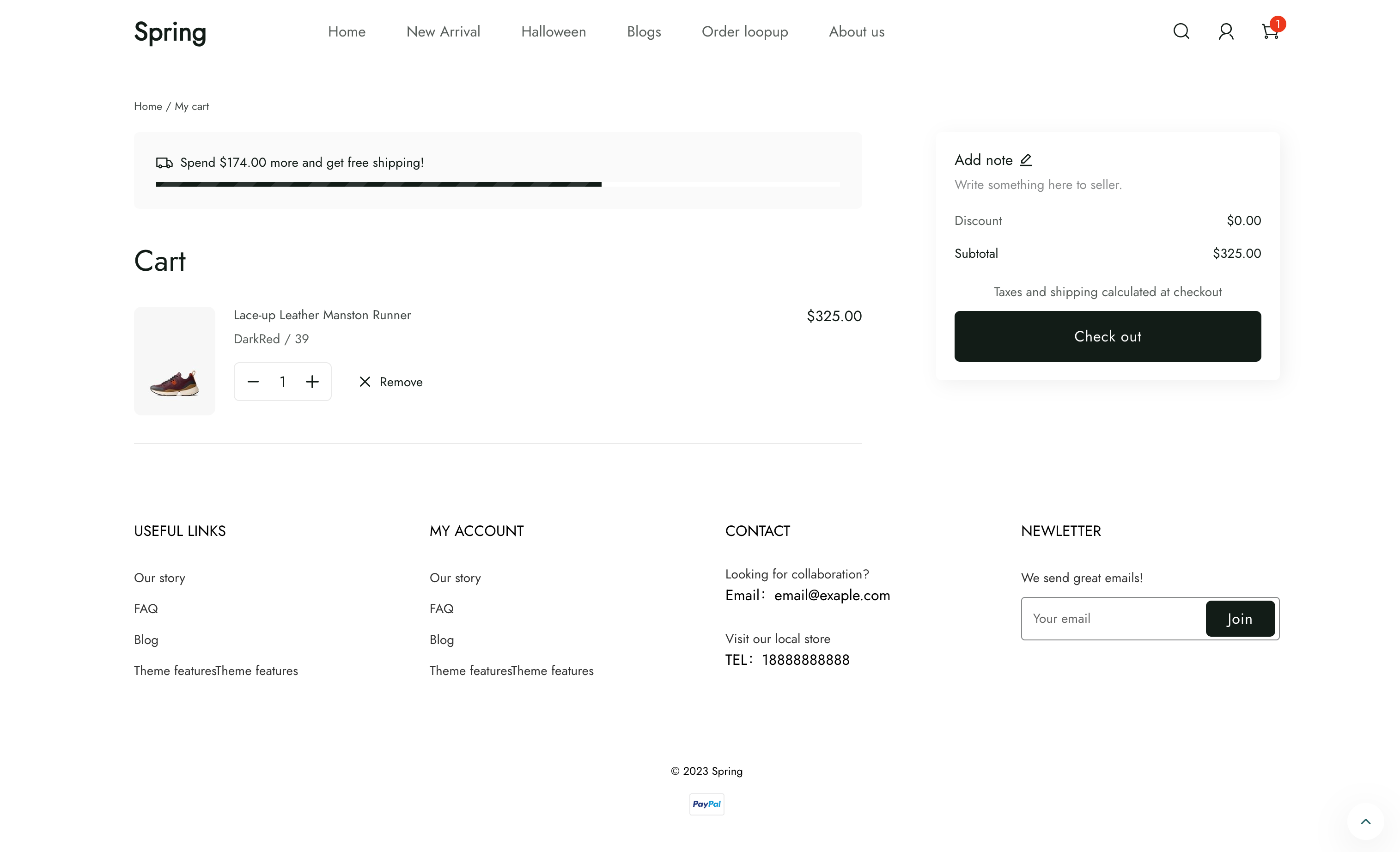
Location
The cart
template is located in the templates
directory of the theme:
└── theme
├── layout
├── templates
| ...
| ├── cart.liquid
| ...
...
Content
You should include the cart object in your section inside the template.
The cart object
You can access the Liquid cart object to display the cart details.
Usage
When working with the cart
template, you should familiarize yourself with the following:
- Using cart line items
- Letting customers proceed to checkout from the cart
- Providing an option to remove line items from the cart
- Updating line item quantities
- Showing discounts
- Using cart notes
- Displaying line item properties in the cart
Cart line items
A line_item is a single line in a shopping cart that records which variant of a product was added, and the associated quantity. For example, if a customer adds both a size medium
and size large
of the same t-shirt
to their cart, then each t-shirt has its own line item.
The cart
template should include a table where each line item has a row:
- The spz-cart component
<spz-cart layout="container">
<template>
<div>
<div spz-for="line_item of data.line_items">
{%- comment -%} line item info {%- endcomment -%}
</div>
</div>
</template>
</spz-cart>
Proceed to checkout
To let customers proceed to checkout from the cart, you need to add a button anywhere, when the button is clicked, call the cart checkout
action to checkout.
<spz-cart id="cart" layout="container">
<template>
<div>
<button type="button" @tap="cart.checkout;">Checkout</button>
</div>
</template>
</spz-cart>
Remove line items from the cart
You should give customers the option to remove a line item from their cart. You need to add a button, and when the button is clicked, call the cart delete
action to remove a line item.
<spz-cart id="cart" layout="container">
<template>
<div>
<div spz-for="line_item of data.line_items">
{%- comment -%} line item info {%- endcomment -%}
<button type="button" @tap="cart.delete(id=${line_item.id})">Remove</button>
</div>
</div>
</template>
</spz-cart>
Tip
Refer to the Cart API to learn more about changing the cart using JavaScript.
Update line item quantities
You should give customers the option to update line item quantities in their cart. You can use spz-quantity component to change quantities.
<spz-cart id="cart" layout="container">
<template>
<div>
<div spz-for="line_item of data.line_items">
{%- comment -%} line item info {%- endcomment -%}
<spz-quantity
value="${line_item.quantity}"
min="1"
max="${line_item.available_quantity}"
layout="flex-item"
height="40"
@quantityChange="cart.update(id=${line_item.id}, value=event.value);"
></spz-quantity>
</div>
</div>
</template>
</spz-cart>
Show cart and line item discounts
Because discounts can apply to an entire cart or to individual line items, you should show discounts with the cart total summary and individual line item displays. To learn more about discounts and how to build discount displays, refer to Discounts.
Support cart notes
You can give customers the option to include additional information with their order through the cart notes.
Cart notes
To capture a cart note, include an HTML input, commonly a <textarea>
, with the attributes name="note"
inside the spz-form component.
<form id="cart-note-form" action-xhr="{{ '/api/cart/note' | add_root_url }}" method="POST">
<textarea
name="note"
@input-debounced="cart-note-form.insert(name='note', value=event.value)"
@change="cart-note-form.submit;"
></textarea>
</form>
Display line item properties
When items are added to the cart, they can have line item properties included with them. You can display these properties by using the parsedProperties
object encapsulated by the component on the cart page by looping through each property:
<spz-cart id="cart" layout="container">
<template>
<div>
<div spz-for="line_item of data.line_items">
{%- comment -%} line item info {%- endcomment -%}
<div spz-for="properties in line_item.parsedProperties">
${properties.name} / ${properties.isImage ? `<a href="${propertie.value}" target="_blank" >View image</a>` : properties.value}
</div>
</div>
</div>
</template>
</spz-cart>
Updated almost 2 years ago